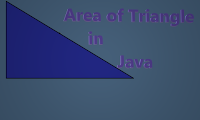
Java Program to find The Area Of a Triangle
This post tell you the way to code a program that can find area of a Triangle.
In different cases when the coordinate of three points are given or the measurement of three sides are given.
(A). Calculating the Area of a triangle when the length of three sides are given
When the length of the three sides are given, we can simply use Hero's
formula to calculate the area.
Java program to find the area of a triangle when the length of three sides are given
import java.util.Scanner; class Main { public static void main(String[] args) { float a, b, c, s; System.out.println("Enter the length of three sides: "); Scanner scan = new Scanner(System.in); a = scan.nextFloat(); b = scan.nextFloat(); c = scan.nextFloat(); s = (a + b + c) / 2; double area = Math.sqrt((s * (s - a) * (s - b) * (s - c))); System.out.println("Perimeter: " + 2 * s); System.out.println("Area: " + area); scan.close(); } }
The output of the Program
Enter the length of three sides: 4 5 6 Perimeter: 15.0 Area: 9.921567416492215
(B). Area of the triangle when the coordinates of vertices are known
If the given coordinates of vertices are (a1, b1), (a2, b2) and (a3, b3)
then the bounded area can be calculated by the formula
[ a1 (b2 - b3) + a2 (b3 - b1) + a3 (b1 - b2) ] / 2
Java Program to find the area of the Triangle having vertices (a1,
b1), (a2, b2) and (a3 , b3)
import java.util.Scanner; class Main { public static void main(String[] args) { float a1, b1, a2, b2, a3, b3; System.out.println("Enter the 3 vertices: "); Scanner scan = new Scanner(System.in); a1 = scan.nextFloat(); b1 = scan.nextFloat(); a2 = scan.nextFloat(); b2 = scan.nextFloat(); a3 = scan.nextFloat(); b3 = scan.nextFloat(); float area = (a1 * (b2 - b3) + a2 * (b3 - b1) + a3 * (b1 - b2)) / 2; System.out.println("Area : " + area + " units"); scan.close(); } }
The output of the Program
Enter the 3 vertices: 0 0 5 0 5 12 Area: 30.0 sq unit