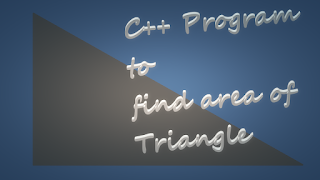
Cpp Program to find the Area of a triangle
This post shows you the way to code a program in cpp that can calulate the area of a triangle.
There are two different cases to calculate area of triangle.
First one when the measurement of sides are known.
Second when the 2d points are given.
Let's code the cpp program for both situation.
(A). Area of the triangle when the length of three sides are given
When the length of three sides are given, we can simply use Hero's formula to
calculate the area of the triangle
C++ program to find the area of the triangle when length of three sides are known
#include <iostream> #include <math.h> // Area = sqrt(s * (s - a) * ( s - b) * (s - c)) // s = (a + b + c) / 2 int main() { float a, b, c; std::cout << "Enter the length of three sides: " << std::endl; std::cin >> a >> b >> c; float s = (a + b + c) / 2; std::cout << "Perimeter: " << 2*s << std::endl; float area = sqrt((s * (s - a) * ( s - b) * (s - c))); std::cout << "Area: " << area; }
The output of the Program
Enter the length of three sides: 4 5 6 Perimeter: 15 Area: 9.92157
(B). Area of the triangle when the coordinates of vertices are known
If the given points are (a1, b1), (a2, b2) and (a3, b3)
then the bounded area can be calculated by the formula
[ a1 (b2 - b3) + a2 (b3 - b1) + a3 (b1 - b2) ] / 2
C++ Program to find the area of the Triangle having points (a1, b1),
(a2, b2) and (a3, b3)
#include <iostream> #include <cmath> int main() { float a1,b1, a2,b2, a3, b3; std::cout << "Enter the 3 vertices: " << std::endl; std::cin >> a1 >> b1; std::cin >> a2 >> b2; std::cin >> a3 >> b3; float area = ( a1* (b2 - b3) + a2 * (b3 - b1) + a3 * (b1 - b2) ) / 2; std::cout << "Area of Triangle: " << std::abs(area) << "sq units"; }
The output of the Program
Enter the 3 vertices: 0 0 5 0 5 12 Area of Triangle: 30sq units