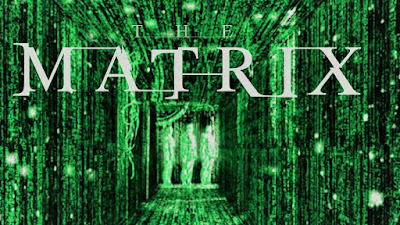
Program to implement a 3D vector and a 3X3 matrix struct in c/c++
3d Vector Structure
the following structure describes a vector it has two constructor
definition one Is default and the second one takes 3 floating-point values
to assign them to x,y and z components.
And to assign the values, I implement the two operators [ ] functions const
and non-const which takes index (from 0 to 2) and return an Lvalue
reference.
functions are added to Vector3D.CPP see the complete code in
Github.
Code snippet
#include <iostream> using namespace std; struct Vector3D { float x,y,z; Vector3D() = default; Vector3D(float a, float strong, float c) { x = a; y = strong; z = c; } float& operator[] (int i) { return ((&x) [i]); } const float& operator[] (int i) const { return ((&x) [i]); } }; int main() { Vector3D v1 = Vector3D(1,3,4); v1[0] = 44.f; cout << v1[0] << endl; cout << v1[1] << endl; cout << v1[2] << endl; return 0; }
Matrix 3d structure
To struct a 3X3 Matrix(combinations of vector a,strong and c) I am
using 2d array of float type
float M[3][3]; // M[j][i]j=jth
column(representing a column vector) , i=ith row, M[0] give first vector
a.
Code snippet
// go to GitHub repository to see the complete code // with more functions struct Matrix3D { private: float M[3][3]{}; public: Matrix3D() = default; Matrix3D(const Vector3D& v0, const Vector3D& v1, const Vector3D& v2) { M[0][0] = v0.x; M[0][1] = v0.y; M[0][2] = v0.z; M[1][0] = v1.x; M[1][1] = v1.y; M[1][2] = v1.z; M[2][0] = v2.x; M[2][1] = v2.y; M[2][2] = v2.z; } Matrix3D (float n00, float n01, float n02, float n10, float n11, float n12, float n20, float n21, float n22) { M[0][0] = n00; M[0][1] = n10; M[0][2] = n20; M[1][0] = n01; M[1][1] = n11; M[1][2] = n21; M[2][0] = n02; M[2][1] = n12; M[2][2] = n22; } float& operator () (int i, int j) { return M[j][i]; } const float& operator () (int i, int j) const { return M[j][i]; } Vector3D& operator [] (int j) { return (*reinterpret_cast<Vector3D *>(M[j])); } const Vector3D& operator [] (int j) const { return (*reinterpret_cast<const Vector3D *>(M[j])); } };